Install extensions
eslint is a linter(a software that highlights errors) and a noder-module package
- eslint
- prettier
Install Eslint globally
npm i -g eslint
and run
eslint --init
- check syntax and find problems
- javascript
- use a popular style guide » Airbnb
Airbnb (https://github.com/airbnb/javascript)
- react
- browser
- js
Bug Workaround:
install errors might happen run the packages individually
npm install eslint-plugin-react --legacy-peer-deps
npm install eslint-config-airbnb --legacy-peer-deps
npm install eslint --legacy-peer-deps
npm install eslint-plugin-import --legacy-peer-deps
npm install eslint-plugin-jsx-a11y --legacy-peer-deps
npm install eslint-plugin-react-hooks --legacy-peer-deps
if it works
a eslintrc.json file is generated. eslint relies on a file called eslintrc to hold rules to check code for errors. run the following to see whether the file has been created
ls -a
Don’t be slow give it a go
run this to see eslint recursively linting all your files
eslint ./src --ext .js
the output is something like this
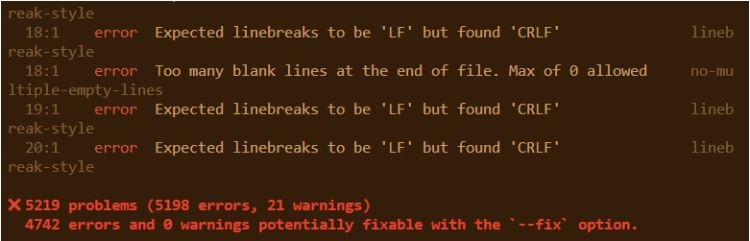
if this command works, we can add the line to “scripts” in the package.json
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject",
"lint": "eslint ./src --ext .js" // this line
},
now we can tryto auto-fix those errors:
npm run lint -- --fix
using eslint and prettier codependently
Note:
prettier unfortunately cannot implement eslint requirements into automated formatting. Prettier relies on a file prettierrc to derive its rules for automated code formatting. In the following we make eslint regard
prettiers rules as correct, so that any rule specified by prettier will not cause a flag with eslint. An example is proposed for the prettierrc.
Steps
install the plugin eslint-config-prettier
npm install --save-dev eslint-config-prettier eslint-plugin-prettier
run the plugin to create the pretierrc at the root directory and link it to the prettier plugin. Do this by executing the command Prettier: Create Configuration File
paste the proposed configuration for prettier:
{
"trailingComma": "es5",
"tabWidth": 2,
"useTabs": false,
"semi": false,
"singleQuote": true
}
add this to elintrc
"extends": ["airbnb", "prettier"],
"plugins": ["prettier"],
"rules": {
"prettier/prettier": ["error"]
},
using eslint and prettier codependently
Note:
prettier unfortunately cannot implement eslint requirements into automated formatting. Prettier relies on a file prettierrc to derive its rules for automated code formatting. In the following we make eslint regard
prettiers rules as correct, so that any rule specified by prettier will not cause a flag with eslint. An example is proposed for the prettierrc.
Steps
install the plugin eslint-config-prettier
npm install --save-dev eslint-config-prettier eslint-plugin-prettier
run the plugin to create the pretierrc at the root directory and link it to the prettier plugin. Do this by executing the command Prettier: Create Configuration File
paste the proposed configuration for prettier:
{
"trailingComma": "es5",
"tabWidth": 2,
"useTabs": false,
"semi": false,
"singleQuote": true
}
add this to elintrc
"extends": ["airbnb", "prettier"],
"plugins": ["prettier"],
"rules": {
"prettier/prettier": ["error"]
},
Hacks
Setting up rules with eslint
under rules we can setup rules.
- 0 turned off
- 1 for warining
- 2 for throwing error
"rules": [
"no-unused-vars" 0 //» tuned off
]
Hacking eslint to make a “dirty deploy”
we can disable all eslint configs, by cleaning the eslintrc.js
module.exports = {
env: {
browser: true,
es2021: true,
},
extends: ['plugin:react/recommended', 'airbnb'], // »» extends: [],
overrides: [],
parserOptions: {
ecmaVersion: 'latest',
sourceType: 'module',
},
plugins: ['react'],
rules: {},
}
this will disable all eslint regulations by circumeventing the linting